What you need to know before Nodejs
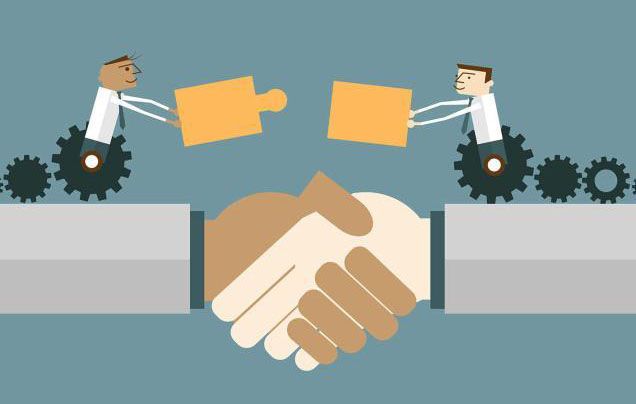
What is Express?
It is a NodeJs framework which provides convenient access to use http, routing, middleware and is perfect to create the API.
What is Middleware?
I would say middleware is a module which is acting like an interceptor. Once client hits your endpoint, it can be either served by the main method and return the response back or can be served by another logic or function which is called middleware. Middleware technically has access to both request and response. In Nodejs we do not have the concept of Asynchronous or threading programming so in order to achieve it, we leverage callback functions! Middleware is basically a layer sitting between request and response expecting some logic.
We actually expect the middleware get the request and send back the response to the next chain otherwise our program will be hanging.
app.use('/vehicleList', function (req, res) {
res.send("response is sent back")
});
Even the function above which is our main function is somehow considered as middleware. So, what express does is just chaining the middleware(s) from request to response. It can also have some logics such as read/write files, connecting to other applications or resources, doing some logics on request or response.
req and res are the common parameters which tell us everything about what tried to access our endpoint and what we will send back as a response to the client.
var cars=['Toyota','Mazda','Subaro'];
var trucks=['Dump','Fuel'];
module.exports.getVehicle= (req,res)=>{
if (req.params.type != 'truck'){
res.json(cars)
}
else{
res.json(trucks)
}
}
app.use('/vehicleList/:type', getVehicle});
in the above examples you already saw the routing in express as well as req/res and middleware.
What are Callbacks and Promises?
Imagine that you called the customer support for your phone call and they hold you on the line for an hour only because they are doing something else and don't have capacity to serve a new customer. What would you expect instead of hanging around? Obviously you would like to leave your number and get a call back when they get free. So, you don't need to be hanging for hours. This is what promises give us. They get the request, prepare the response and once they are ready, then will tap on your shoulder and tell you if they successfully provided the response or it is failed.
With this callbacks we basically implement Asynchronous programming while sometimes we need to make sure that we definitely have the result when we are executing the next command. We can implement it with lots of nested call backs to get the result and pass to the next call back but we call it callback hell which makes the programming really complicated. However, we need a safe method that promise us we will get the result while we avoid the complexity.
Promises:
When you order a sandwich, they will give you an order number which is a reciept and basically a placeholder for your sandwich. Once your turn is coming, you only have two options:
-
you will get your sandwich (Fulfill)
-
you will get an excuse that the cafe ran out of bread and there is no sandwich for today (Reject)
It means that our promise object which is returned from a function has different states. Recipient can subscribe to the function when it is done and see if the result is reject or fulfil. multiple promises can be chained up. It means that in the fulfill function we can return second promise. So, we do not have nested callbacks any more although we have all functions in a vertical chain. But most importantly is that we leverage a safety and trust that we definitely have the response when we are invoking the next function.
Using Native Promises of Javascript or 3th parties libraries ?
Bluebird for promises:
Bluebird is a library which is compatible with ES6 and works well with v8 which is a engine behind chrome and other modern browsers.
In fact instead of having a callback as a parameter in our function, we chain our method with then or reject or other method of bluebird. We even can convert some modules like fs.readfile module to Asynchronous module with help of bluebird.
npm i bluebird --save
How Node loads different modules in a right order?
in order to access the modules either from node_module or another js file we need a module loader. Node uses CommonJs with the following syntax under the hood.
// loading a 3d party library installed by npm
var promise= require('bluebird')
//loading another js file fron our project
var handler= require('./handler')
//loading build in modules of node
var fs= require('fs');