Setup DEV box for existing Sitecore project
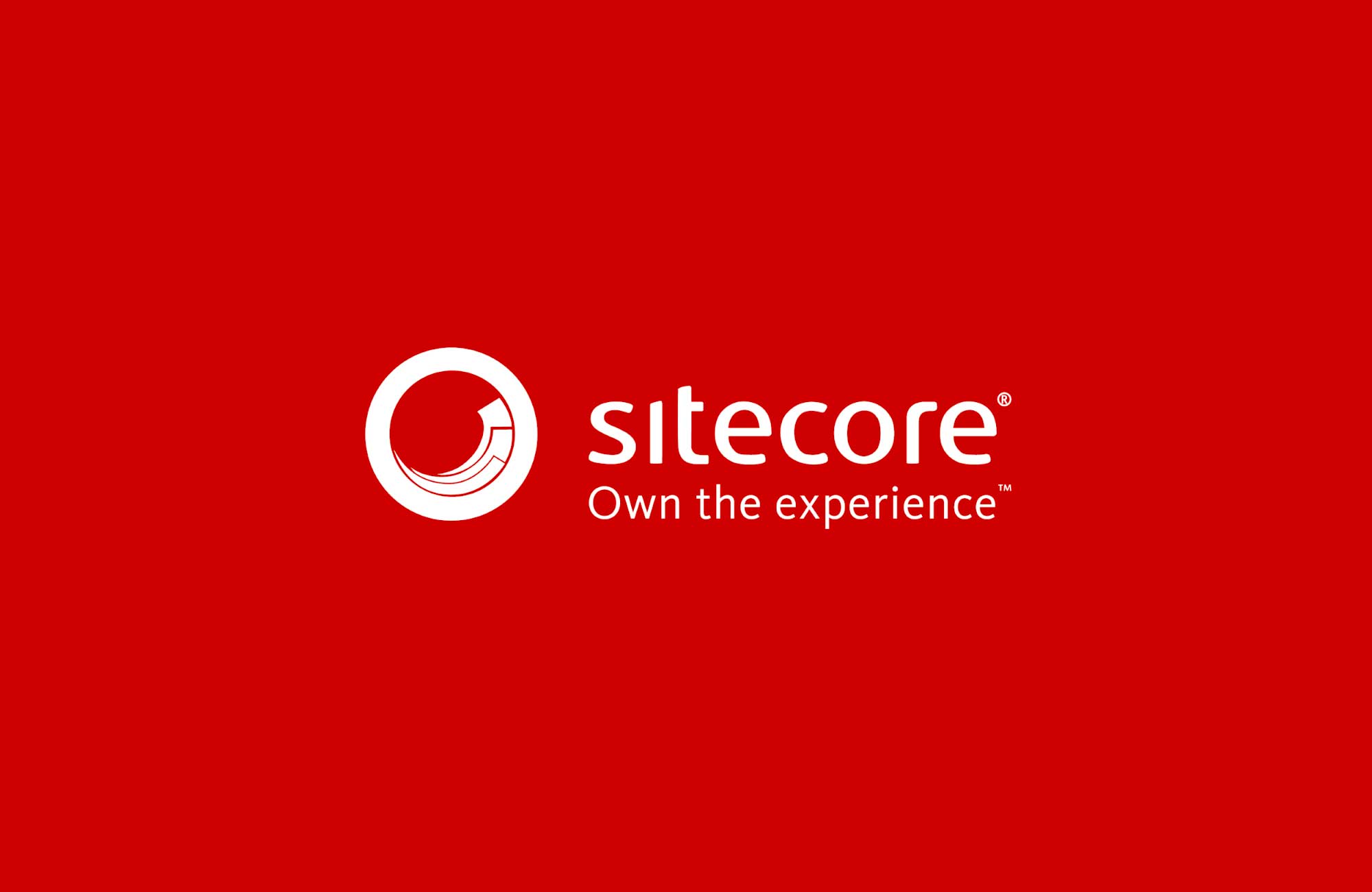
Setup sitecore project is really easy with SIM. However, sometimes you cannot use it due to network security issue or other reasons. Then you have to setup everything manually.
Following the steps below might help.
Install Supporting applications:
- Local IIS Server
- Sitecore Rocks [optional]:
Sitecore Rocks provides a convenient way to access your Sitecore instance from Visual Studio and is required by TDS.
steps:- Open up Visual Studio
- Select Tools -> Extensions and Updates.
- Select “Online” to the left
- Search for “Sitecore”
- Select Sitecore Rocks from the list and click Download
- Follow the steps in the installer
- Restart Visual Studio.
- Sitecore Rocks will then ask you to install the Template Wizard. This is an optional component, feel free to install it as you see fit.
- SQL Server (express is ok)
- Visual Studio
- GIT
- Node and Grunt: Setup for Node, Grunt & Visual Studio 2015
- Hedgehog TDS
steps:
-
TDS enables developers to sync database changes to a (source controlled) file system.
-
Download the latest version here
-
Unzip and run the install for your version of Visual Studio
Agree,Next, Next, Next
Enter Licence Key
NOTE: Re. error "The version of the sitecore connector is from a different version of TDS", you should check:
The TDS output window - it will tell you which version you're running and the version that's in the website folder
You're running the correct version of TDS
- Remove the _DEV folder from the website direction
- Remove the HedgehogDevelopment.SitecoreProject.Service.dll from the Website\bin folder
- Re-run Publish Local
Steps
clone the repository of your visual studio code
Setup IIS bindings
you can run the function nelow in your powershell script:
function ConfigureSiteCore {
1. Install WebPI
cinst webpi
WebPiCmd /Install /AcceptEula /Products:ARRv3_0
WebPiCmd /Install /AcceptEula /Products:UrlRewrite2
$bindings = @(
"www.training.MySite.local",
"www.sell.MySite.local"
)
2. Create web site and app pool
Write-Host "Create App Pool and Sitecore site"
$appPool = "MySiteAppPool"
CreateAppPool $appPool
Run command:
New-WebSite -Name "MySite" -Port 80 -PhysicalPath "$env:RootCodeFolder\Dev\SiteCore\Website" -ApplicationPool $appPool -IPAddress "*" -HostHeader MySite
Run command:
New-WebBinding -Name "MySite" -IPAddress "*" -Port 443 -Protocol https -HostHeader MySite
3. Add bindings
foreach ($binding in $bindings) {
New-WebBinding -Name "MySite" -IPAddress "*" -Port 80 -Protocol http -HostHeader $binding
New-WebBinding -Name "MySite" -IPAddress "*" -Port 443 -Protocol https -HostHeader $binding
}
}
Install MongoDB
you can run the function nelow in your powershell script:
function InstallMongoDB {
1. MongoDB Install
cinst mongodb
2. Grab the recently installed Mongo version and setup paths
$mongoPath = "C:\Program Files\MongoDB\Server"
$mongoVersion = (Get-ChildItem "$mongoPath" | ?{ $_.PSIsContainer } | Select-Object Name | Sort-Object | Select-Object -First 1).Name
$mongoBinPath = "$mongoPath\$mongoVersion\bin"
$mongoLogsPath = "$env:RootDatabaseFolder\mongodb\log"
$mongoDataPath = "$env:RootDatabaseFolder\mongodb\db"
3. Create data dirs
New-Item -ItemType Directory -Force -Path "$mongoLogsPath"
New-Item -ItemType Directory -Force -Path "$mongoDataPath"
4. Create Config
@"
systemLog:
destination: file
path: $mongoLogsPath\mongod.log
storage:
dbPath: $mongoDataPath
"@ | Out-File -FilePath "$mongoBinPath\mongod.cfg"
5. Install
&"$mongoBinPath\mongod.exe" --config "$mongoBinPath\mongod.cfg" --install
Start-Service MongoDB
}
Restore Databases
This step will populate the databases with the most up to date data
- Stop the MySite website
- Request\Retrieve a backup of the Sitecore databases
you can use this script:
USE [master]
GO
CREATE PROCEDURE #backupdb (@dbname AS NVARCHAR(300))
AS
BEGIN
DECLARE @date NCHAR(8) = CONVERT(VARCHAR(10), CURRENT_TIMESTAMP, 112)
DECLARE @drop NVARCHAR(300) = 'E:\Drop\' + @date
DECLARE @path AS NVARCHAR(300) = @drop + '\'+@dbname+'.bak'
EXEC master.dbo.xp_create_subdir @drop
PRINT 'Backing up ' + @dbname + ' to ' + @path
BACKUP DATABASE @dbname TO DISK = @path
PRINT @dbname + ' Backed up'
END
GO
EXEC #backupdb 'MySiteSitecore_Analytics'
EXEC #backupdb 'MySiteSitecore_Core'
EXEC #backupdb 'MySiteSitecore_Master'
EXEC #backupdb 'MySiteSitecore_Sessions'
EXEC #backupdb 'MySiteSitecore_Web'
EXEC #backupdb 'MySiteSitecore_Wffm'
PRINT 'All Databases Backed Up'
DROP PROCEDURE #backupdb
GO
- Restore the backups locally
you can use this script:
USE [master]
GO
CREATE PROCEDURE #restore (@dbname AS NVARCHAR(300))
AS
BEGIN
DECLARE @date NVARCHAR(8) = CONVERT(VARCHAR(10), CURRENT_TIMESTAMP, 112) --This is today
-- Change these values if you need to pick backups
-- from another location or you don't want them to
-- go to the default location
DECLARE @backup_template NVARCHAR(MAX) = N'E:\Drop\{date}\{dbname}.bak'
DECLARE @data_template NVARCHAR(MAX) = CONVERT(NVARCHAR(MAX),SERVERPROPERTY('InstanceDefaultDataPath')) + N'{dbname}.mdf'
DECLARE @log_template NVARCHAR(MAX) = CONVERT(NVARCHAR(MAX),SERVERPROPERTY('InstanceDefaultLogPath')) + N'{dbname}_log.ldf'
DECLARE @logname AS NVARCHAR(300) = @dbname + '_Log'
DECLARE @backup_path AS NVARCHAR(300) = REPLACE(REPLACE(@backup_template, '{date}', @date), '{dbname}', @dbname)
DECLARE @data_path AS NVARCHAR(300) = REPLACE(@data_template, '{dbname}', @dbname)
DECLARE @log_path AS NVARCHAR(300) = REPLACE(@log_template, '{dbname}', @dbname)
DECLARE @old_data_name AS NVARCHAR(MAX)
DECLARE @old_log_name AS NVARCHAR(MAX)
-- This extracts the database name from the backup just in case they are different
--RESTORE FILELISTONLY FROM DISK = @backup_path
DECLARE @BackupFiles TABLE(LogicalName nvarchar(128),PhysicalName nvarchar(260),Type char(1),FileGroupName nvarchar(128),Size numeric(20,0),MaxSize numeric(20,0),FileId tinyint,CreateLSN numeric(25,0),DropLSN numeric(25, 0),UniqueID uniqueidentifier,ReadOnlyLSN numeric(25,0),ReadWriteLSN numeric(25,0),BackupSizeInBytes bigint,SourceBlockSize int,FileGroupId int,LogGroupGUID uniqueidentifier,DifferentialBaseLSN numeric(25,0),DifferentialBaseGUID uniqueidentifier,IsReadOnly bit,IsPresent bit,TDEThumbprint varbinary(32));
INSERT @BackupFiles EXEC('RESTORE FILELISTONLY FROM DISK ='''+ @backup_path+'''');
SELECT @old_data_name = LogicalName FROM @BackupFiles WHERE Type = 'D';
SELECT @old_log_name = LogicalName FROM @BackupFiles WHERE Type = 'L';
PRINT 'Restoring ' + @old_data_name + ' as ' + @dbname + ' from ' + @backup_path + ' into ' + @data_path
RESTORE DATABASE @dbname FROM DISK = @backup_path
WITH FILE = 1,
MOVE @old_data_name TO @data_path,
MOVE @old_log_name TO @log_path,
NOUNLOAD, STATS = 5
PRINT @dbname + ' Restored'
END
GO
EXEC #restore 'MySiteSitecore_Analytics'
EXEC #restore 'MySiteSitecore_Core'
EXEC #restore 'MySiteSitecore_Master'
EXEC #restore 'MySiteSitecore_Sessions'
EXEC #restore 'MySiteSitecore_Web'
EXEC #restore 'MySiteSitecore_Wffm'
PRINT 'All Databases Restored'
DROP PROCEDURE #restore
GO
- Run the script below to set the permissions for your account in SQL:
USE [master]
GO
DROP LOGIN [SiteCoreDeveloper]
GO
GO
CREATE LOGIN [SiteCoreDeveloper] WITH PASSWORD=N'SiteCoreDeveloper', DEFAULT_DATABASE=[master], DEFAULT_LANGUAGE=[us_english], CHECK_EXPIRATION=OFF, CHECK_POLICY=OFF
GO
USE [MySiteSitecore_Analytics]
GO
DROP USER [SiteCoreDeveloper]
GO
CREATE USER [SiteCoreDeveloper] FOR LOGIN [SiteCoreDeveloper]
ALTER ROLE [db_owner] ADD MEMBER [SiteCoreDeveloper]
GO
USE [MySiteSitecore_Core]
GO
DROP USER [SiteCoreDeveloper]
GO
CREATE USER [SiteCoreDeveloper] FOR LOGIN [SiteCoreDeveloper]
ALTER ROLE [db_owner] ADD MEMBER [SiteCoreDeveloper]
GO
USE [MySiteSitecore_Master]
GO
DROP USER [SiteCoreDeveloper]
GO
CREATE USER [SiteCoreDeveloper] FOR LOGIN [SiteCoreDeveloper]
ALTER ROLE [db_owner] ADD MEMBER [SiteCoreDeveloper]
GO
USE [MySiteSitecore_Sessions]
GO
DROP USER [SiteCoreDeveloper]
GO
CREATE USER [SiteCoreDeveloper] FOR LOGIN [SiteCoreDeveloper]
ALTER ROLE [db_owner] ADD MEMBER [SiteCoreDeveloper]
GO
USE [MySiteSitecore_Web]
GO
DROP USER [SiteCoreDeveloper]
GO
CREATE USER [SiteCoreDeveloper] FOR LOGIN [SiteCoreDeveloper]
ALTER ROLE [db_owner] ADD MEMBER [SiteCoreDeveloper]
GO
USE [MySiteSitecore_Wffm]
GO
DROP USER [SiteCoreDeveloper]
GO
CREATE USER [SiteCoreDeveloper] FOR LOGIN [SiteCoreDeveloper]
ALTER ROLE [db_owner] ADD MEMBER [SiteCoreDeveloper]
GO
Install local sitecore instance
- Download
Sitecore 8.1 rev. 160302
from sitecore site - Unzip the entire folder
- Run 'Sitecore 8.1 rev. 151003.exe" locally as admin????????????
Instance Name: MySite - This name will be used for the website in IIS, the database prefixes, the root folder, the host name, etc. - Point the installer to "license.xml" you copied in step 2
- Enter your local database details
Install Sitecore in "D:\Dev\mysite\SiteCore" (or wherever your repository is cloned)
Web Site name: MySite
Finsh!
Open http://training.MySite/sitecore
Login with
username: admin|xxx
password: b|xxx
Move the Data directory
cIn order for the relative data folder to work in Sitecore it’s easiest to put the /data folder under /website
Stop the MySite Website and AppPool
Move D:\Dev\mysite\SiteCore\Data folder into D:\Dev\mysite\SiteCore\Website
Start the MySite Website and AppPool
Copy D:\Dev\mysite\SiteCore\Website\Data\licence.xml to D:\Dev\mysite\SiteCore\Data\licence.xml or if you got error create another licence folder then paste the file.
Edit host file
edit the hosts file in C:\Windows\System32\drivers\etc
and add the bindings which you have also in IIS.
IIS features
you need heaps of IIS and windows features like:
Import-Module ServerManager
Add-WindowsFeature -Name Web-Default-Doc, Web-Dir-Browsing, Web-Http-Errors, Web-Static-Content, Web-Http-Logging, Web-Stat-Compression, Web-Dyn-Compression, Web-Filtering, Web-Asp-Net45, Web-Mgmt-Console
which might not work in windows 7. But you can use equal commands like:
# IIS
choco install --source windowsfeatures IIS-WebServerRole
choco install --source windowsfeatures IIS-WebServerManagementTools
choco install --source windowsfeatures IIS-NetFxExtensibility
choco install --source windowsfeatures IIS-ISAPIExtensions
choco install --source windowsfeatures IIS-ISAPIFilter
choco install --source windowsfeatures IIS-ASPNET
choco install --source windowsfeatures IIS-DefaultDocument
choco install --source windowsfeatures IIS-DirectoryBrowsing
choco install --source windowsfeatures IIS-HttpErrors
choco install --source windowsfeatures IIS-HttpRedirect
choco install --source windowsfeatures IIS-StaticContent
choco install --source windowsfeatures IIS-HttpLogging
choco install --source windowsfeatures IIS-RequestMonitor
choco install --source windowsfeatures IIS-HttpTracing
choco install --source windowsfeatures IIS-ManagementService
choco install --source windowsfeatures IIS-ManagementScriptingTools
choco install --source windowsfeatures IIS-HttpCompressionStatic
choco install --source windowsfeatures IIS-RequestFiltering
choco install --source windowsfeatures IIS-WindowsAuthentication
Note: Install Chocolatary with Brew in your machine.
Publish solution
Open the Visual studio solution which you cloned.
Restore the Nuget packages and run the build.
You need to deploy you application to the sitecore website you created and connected to IIS.
you can use a full script or alternatively do some of them manually and only run the next script:
# These params are named params and are passed into the script from the command line.
# "D:\dev\mysite\SiteCore\Website"
param(
[Parameter(Mandatory=$false)]
$SolutionDir="D:\Dev\mysite",
[Parameter(Mandatory=$false)]
$SolutionFileName="MySite.Sitecore.sln",
[Parameter(Mandatory=$false)]
$ShouldClean,
[Parameter(Mandatory=$false)]
$scSitePath="D:\Dev\mysite\SiteCore\Website",
[Parameter(Mandatory=$false)]
$scInstallPath = "D:\dev\Sitecore8.1rev.160302\Website"
)
function Get-ScriptDirectory{
$Invocation = (Get-Variable MyInvocation -Scope 1).Value
Split-Path $Invocation.MyCommand.Path
}
function CleanBin($dirPath){
if([System.IO.Directory]::Exists($dirPath)){
Write-Host "clean path $dirPath"
Get-ChildItem -Path $dirPath -Filter "mysite*, MySite*" | foreach { BruteForceRemove $_ }
}
}
# There exists a problem withm Remove-Item -recurse -force. It doesn't always work, and the "solution"
# is to repeat the operation until the folder has been cleared.
function BruteForceRemove($dirPath) {
$iteration = 0
Write-Host "BruteForceRemove $dirPath"
while ( $iteration++ -lt 10)
{
if (Test-Path $dirPath)
{
Remove-Item $dirPath -recurse -force -EA SilentlyContinue
}
else
{
Write-Host "$dirPath deleted in $iteration iterations"
break
}
}
}
Write-Host "@@@@@ Begin local publish @@@@@"
$tdsConnectorInstallPath = "$SolutionDir\Build\_DEV"
$elapsed = [System.Diagnostics.Stopwatch]::StartNew()
write-host "Started at $(get-date)"
Write-Host "Are we cleaning? $ShouldClean"
Write-Host "Solution directory $SolutionDir"
Write-Host "Solution filename $SolutionFileName"
Write-Host "Sitecore Website Root Path $scSitePath"
Write-Host "Sitecore Clean Install Path $scInstallPath"
Write-Host Killing W3s...
Get-Process *w3* | Stop-Process -Force
if($ShouldClean){
BruteForceRemove $scSitePath;
Write-Host "About to copy the base Sitecore install";
XCOPY $scInstallPath $scSitePath\ /sy
} else{
CleanBin "$scSitePath\bin";
}
$buildTarget = if($ShouldClean) { "rebuild" } else { "build" };
Write-Host "The build target for MSBUILD is $buildTarget"
$msbuildExe = "C:\Program Files (x86)\MSBuild\14.0\Bin\msbuild.exe";
$args = "$SolutionDir\$SolutionFileName /t:$buildTarget /p:DeployOnBuild=true;PublishProfile=LocalPublish /verbosity:normal"
$process = Start-Process -NoNewWindow -FilePath $msbuildExe -PassThru -Wait -ArgumentList $args;
$aol=Invoke-WebRequest https://www.training.MySite.local/
Write-Host "@@@@@ End local publish @@@@@"
write-host "Ended at $(get-date)"
write-host "Total Elapsed Time: $($elapsed.Elapsed.ToString())"
exit 0;
Or simply run the command in the solution root (ex- D:\Dev\mysite) below specially if you already created the publish profile
:
"C:\Program Files (x86)\MSBuild\14.0\Bin\msbuild.exe" %1 /p:DeployOnBuild=true;PublishProfile=LocalPublish