Hybrid solution with Typescript, Vue components and ES2015+
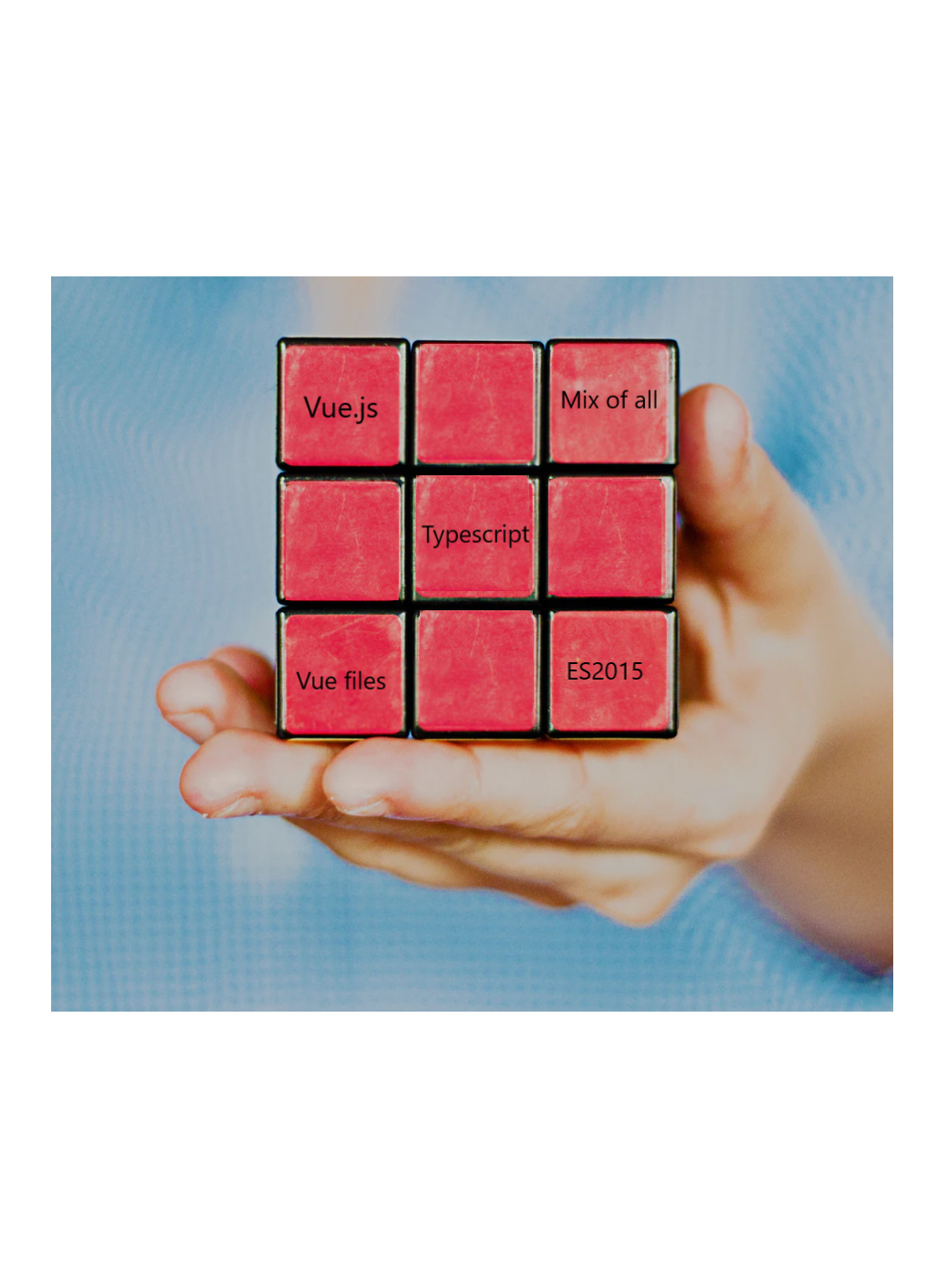
Vue version 3 is written by Typescript and quite compatible with it but officially I am still using Vue version 2 which is not 100% aligned with Typescript.
There are a few approaches for Vue version 2 depends on how much you are lookingg to use Typesript in your project and wheather it is an existing legacy project or new green field.
I am discussing them here in this post that might help you finding a clue and going further from here.
First Approach : Adding typescript using Vue plugin
Adding typescript into existing project will add all dependencies and convert all files to be TypeScript compatible. which mean you have to do heavy regression testing as it will convery all .vue
files to .ts
In order to add it to the new project, you simply ask Vue cli to include Typescript but for adding it to an existing project you just need to run this command:
Vue add Typescript
Once it is done succesfully, it will ask a few questions and will convert all babel transpiler, linter and Vue components and js files to ts;
which was not ideal for me since running the project will have a lot of breaking parts.
All files are touched
Second Approach: Adding typescript manually
When I post my question in Twitter as below, some of the freinds told me you can simply add Typescript files and import them and it should work. But I figured out it needs some step to be taken for me to add my ts files.
I need a good source to see how can I mix typescript with JavaScript in vuejs. Still want to keep vue components in ES6+ but import some typescript files into my component. Any idea ?? Most resources show how to convert vue components to typescript #vuejs #TypeScript @vueconf
— Nelly Sattari (@nelly_sattari) June 2, 2020
Obviously you can go ahead and create your new .ts
file without adding any library to the project. Then you import it to your component.
But once you run your project , you will get error that you don’t have ts-loader
In order to fix it, You need to run this command:
npm i -D typescript ts-loader
Then you add this bit to the vue.config to introduce a new ts-loader to our webconfig.js
config.module.rules.push({
test: /\.(ts)$/,
exclude: /node_modules/,
loader: 'ts-loader',
});
Also you need to at this line to the same file:
config.resolve.extensions.push('.ts');
tsconfig.json
file is essential to transpile Typescript to Javascript which should be created at the root of the project.
{
"compilerOptions": {
"lib": ["dom", "es5", "es2015"],
"target": "es5",
"module": "es2015",
"moduleResolution": "node",
"sourceMap": true,
"allowSyntheticDefaultImports": true
}
}
Everything works now but you don’t have any Typescript intelligence as part of your project.
Other approaches and questions
There are some questions and further investigations that I didnot cover as part of this post:
- Some people believe in order to get proper annotation and intelligence the approach above cannot help and we need to go with third approach which is using vue-class-component
This means we need to write our vue component in the format of generic class not the way we write it currently.
Some other believe this is not a way for Vue company to go forward and might be deprecated. - How we get one Lint to compile both Js and TS files. Currently we have Eslint but in order to compile ts files we need TsLint .