JSS, THE HEADLESS CMS- PART2
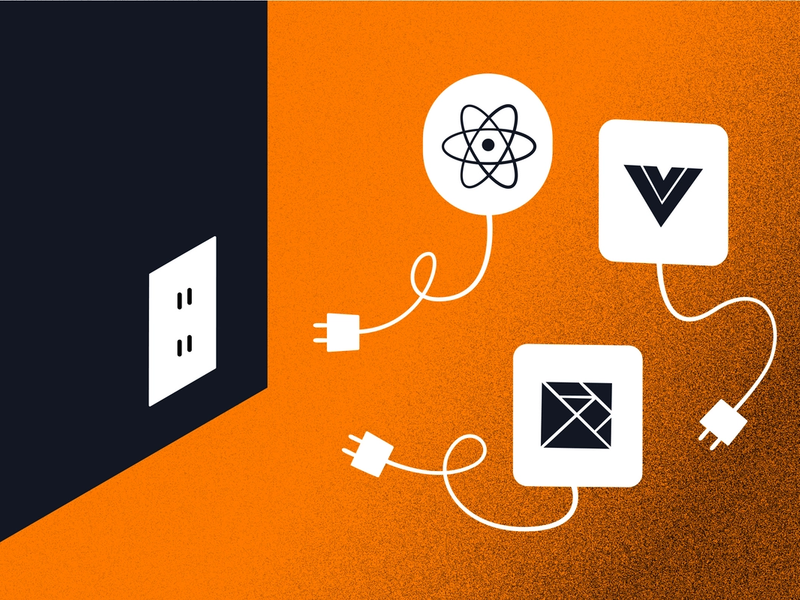
In this post, I will show how I am using JSS to build a dynamic frontend app with reusable components. Also how to build a new web app with Vuejs and JSS which is content editable and dynamic.
For what use case I am using JSS?
I need to design a web app which has so many reusable components with different contents and routing. What solution do you think of having these two features, content editable and dynamic routing:
- Web app should be content editable means all images, button titles, labels and ... should be editable by content authors.
- Web app should be smart enough to reuse the components and route to different pages based on which screen is active.
Example as below:
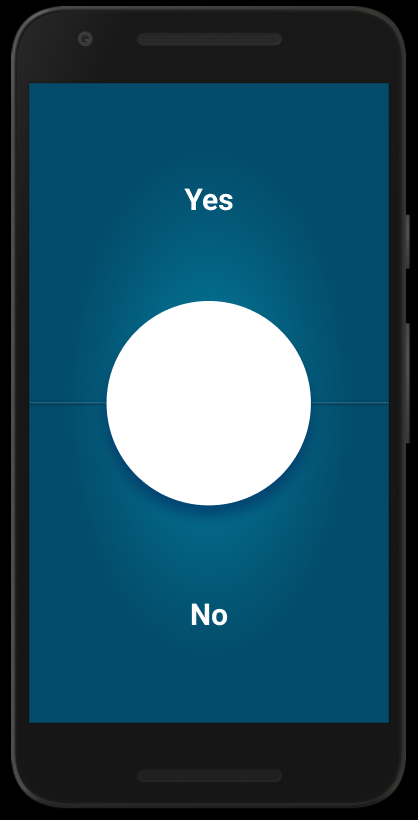
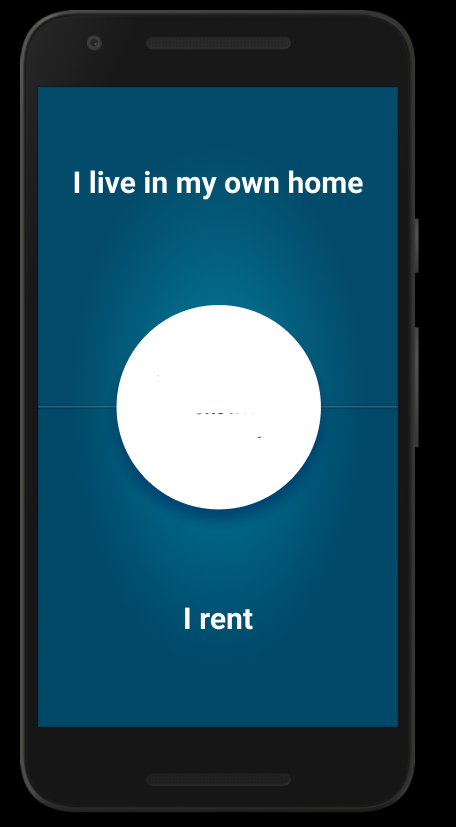
How JSS can help?
JSS is basically the JavaScript SDK which is built by Sitecore. You can the most out of JSS if you use Sitecore as a CMS content management service, however, for simple scenarios you can use JSS independent from Sitecore and use it in headless mode getting data from any platform that understands JSON.
In this post, I will show you how to use JSS in development mode with no need to have sitecore installed on your machine.
Disconnected developer mode
With disconnected mode, content data is mocked using local files (json/yaml/js) instead of a Sitecore instance. Obviously, you can later on import your app to the Sitecore when you decided to use it.
Build our first component with Vue.js and JSS
First all all in order to install JSS and create your application you need to look at my another post or JSS documentation.
Now that your app is up and running, let's build your first component with dynamic content and route.
jss scaffold <ComponentName>
Example: jss scaffold Disclaimer
Component name should start with Capital letter
what this command does is creating two files for you:
- src/components/Disclaimer.vue
This file is your simple Vue component with Html and Script
<template>
<div>
<p>Disclaimer Component</p>
<sc-text :field="fields.heading" />
</div>
</template>
<script>
import { Text } from '@sitecore-jss/sitecore-jss-vue';
export default {
name: 'Disclaimer',
components: {
ScText: Text,
},
props: {
fields: {
type: Object,
default: () => ({}),
},
},
}
</script>
A few extra lines to a vue components are there which is all about using pre-defined Sitecore components like Textbox, Rixhtextbox, Images ,...
import { Text } from '@sitecore-jss/sitecore-jss-vue';
<sc-text :field="fields.heading" />
This is a text box component where you import and you can either use it or use your own component.
3. sitecore/definitions/components/Disclaimer.sitecore.js
There is a Sitecore folder associated to each newly created component which holds the data schema for the component. These are the data fields and Sitecore configuration for the component.
The content of this file looks like below:
import { CommonFieldTypes, SitecoreIcon, Manifest } from '@sitecore-jss/sitecore-jss-manifest';
/**
* Adds the Disclaimer component to the disconnected manifest.
* This function is invoked by convention (*.sitecore.js) when 'jss manifest' is run.
* @param {Manifest} manifest Manifest instance to add components to
*/
export default function(manifest) {
manifest.addComponent({
name: 'Disclaimer',
icon: SitecoreIcon.DocumentTag,
fields: [
{ name: 'heading', type: CommonFieldTypes.SingleLineText },
],
});
}
Basically it describes the dynamic fields for this component and their type.
Add your first component to the Route and pass content dynamically
While you are usinf disconnected mode, you can rely on a mock yml file with all content you need.
- Open /data/routes/en.yml. file and you can add your component to this route which basically the home page.
Now when you run jss start
you will see the Disclaimer
component added to the home page.
Add your component to another page
If you want to add your component to another page like disclaimer page
with maybe different content you can create another yml file inside a new folder as below:
Then when you navigate to the disclaimer page
you will see your component added to this page with different contents from homepage.
under the hood
Once you run your app basically your JSS send a HTTP request to get the content based on what mode your are running your app.
When you run your app in disconnected mode
, JSS get the routes and contents and schema from your mock yml files. However, if you use connected mode, JSS send the request to the Sitecore layout service and retrieve the content from Sitecore which I will show you in another post.
Conclusion
That is how you can build one component but pass different content to it to render it dynamically based on the content file. So you basically can reuse your component to be rendered differently and still is reusable.
In the next post I will show you how to import your JSS into Sitecore and basically drive the content from sitecore instead of your mock yml file.