Git Tricks
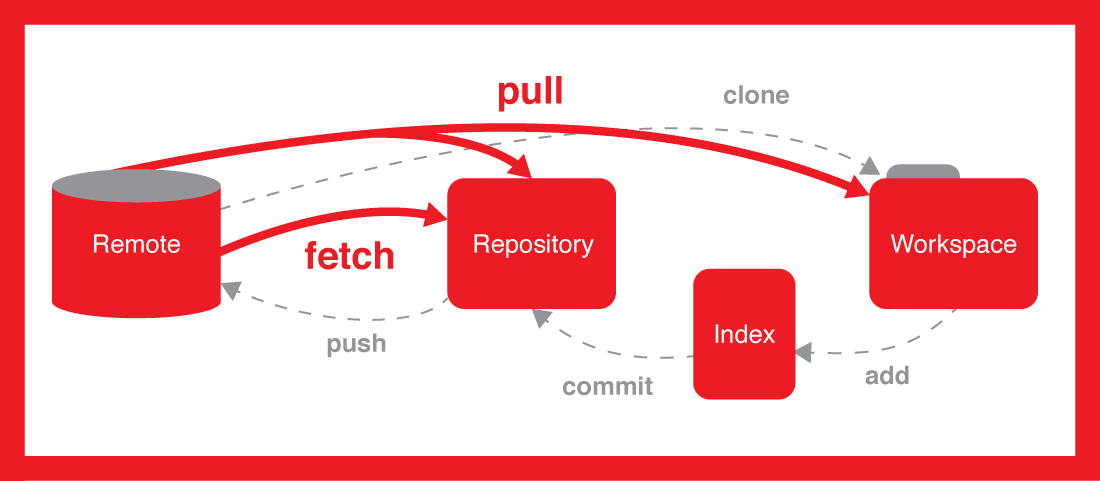
Git Fork
If you are one of the people who use fork you need to know some concepts and commands per below. No doubt Fork is much cleaner way of code management.
How to fork?
Simply go to the repo and click fork button
What is happening after fork?
In fact you will have two downstream (origin) and upstream.
Origin in the version of code in your local and upstream is the actual original code.
Once you changed code in your local, you first need to push it to your origin and then you will send a pull request to the upstream for getting merged.
Basiacally, there are sometimes that you need to sync your local (origin) repo with the upstream too. Assuming that we now how to push and send PR to upstream since it is exactly like the normal way of cloning. You can find the other scenarios though in below.
How to sync your local repo with upstream?
You want to know where you are first:
git remote -v
SO it will show you the origin and upstream. Origin in your local repo and upstream is the repo you forked your repo from
First yuou need to introduce which repo is your upstream
git remote add upstream git@github.com:xx/xx.git
Then you need to get all the branches in your local:
git fetch upstream
when you do a fetch it is just syncing the history and branches. If you want the updated code into your nranch, you need to pull it as well:
You will see the update after this command. Make sure you are in the right branch.
git checkout master
git pull
It seems other than master
, you cannot checkout to any other branches!!!!
checkout a new branch to track the upstream branches
First you can see what branches are available :
git branch -v -a
Then you will create a new branch from the upstream branch which also tracks that upstream
git checkout -b releases --track origin/releases
Sync your upstream into the origin
First you need to fetch the upstream
git fetch
Then you need to pull the latest changes
git checkout releases
git pull
Now you should merge the upstream with your origin branch
git merge upstream/releases
It is ready so you can push the new changes to your origin branch
git push
Apply the recent code from upstream to your origin repo:
If you want to move all your history use:
git rebase upstream/master
Otherwise use :
git merge upstream/master
Probably you need to push and update your origin local repo if there are any changes
git push origin master
Push your local changes to the upstream
Obviously normal push will push your changes to Origin/local then you can send a pull request to merge and push your changes to the upstream.
you also can use:
git push upstream
if the project is yours and does not need pull request.
Point your repo to other repos
Somehow you decide to change your repo address and point it to the other urls.
git remote set-url origin git@github.com:yourforkedname/xxx.git
git remote set-url upstream git@github.com:youroriginalname/xxx.git
Git clean
There is a repo which has folder A
in master branch. Once I switch to test branch
which does not have folder A
, I still can see the folder which means it is left over from the other branch and for some reasons it did not overrided.
using this command will clean up the untracked files in your branch.
git clean -fdx
How to revert just a few files in a commit and keep others manually
Find the clean commit that you messed up in that. You want to go one step back so you just need to say which files you want to revert then run this command:
In the following example commitId is HASHfbdea
. The sign ~1 means go one step before my commit ID:
Format is:
git checkout commitId~1 FilePath
Example:
git checkout HASHfbdea~1 main/cotent/help.css
How to revert just a few files in a commit and keep others with Git extension
Git extension=> see each commit one by one and see the files you dont want them. select them and press right click on the files you dont want them and revert to parent then in your local stage and commit them
Rename a local and remote branch in git
If you have named a branch incorrectly AND pushed this to the remote repository follow these steps before any other developers get a chance to jump on you and give you shit for not correctly following naming conventions.
- Rename your local branch.
If you are on the branch you want to rename:
git branch -m new-name
If you are on a different branch:
git branch -m old-name new-name
- Delete the old-name remote branch and push the new-name local branch.
git push origin :old-name new-name
- Reset the upstream branch for the new-name local branch.
Switch to the branch and then:
git push origin -u new-name