JSS,THE HEADLESS CMS- PART 3 Style your Vue JSS with Vuetify
Styling your JSS Vue.js app with using Vuetify a library based on Material Design
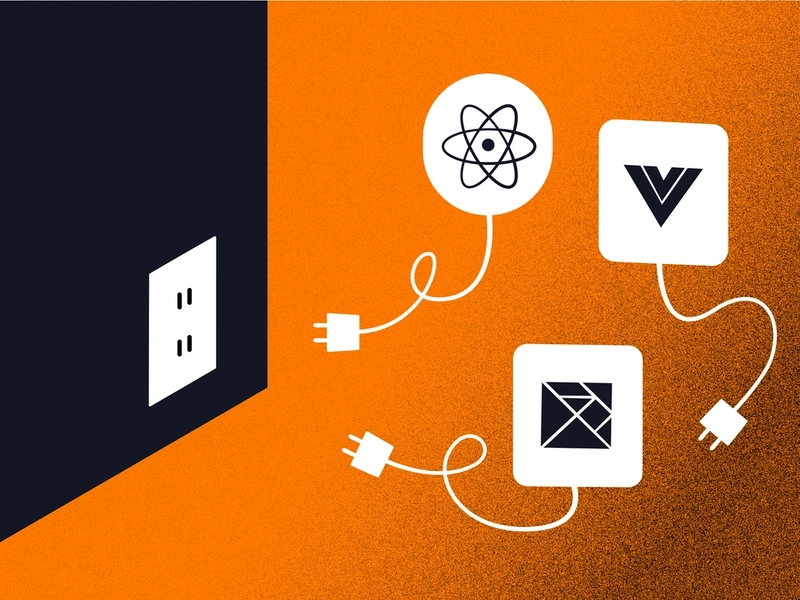
While some statistics show that using utility based CSS is more popular according to this link , still some people prefer to use a framework based on Material Design or Bootstrap or others to be aligned with their UX designers. In This post the focus is on Vuetify specifically using it with JSS
Vuetify is based on Material Design
If you use Vue
and prefer to use Material Design then maybe go for Vuetify
!
Vuetify is the component library for Vue.js and has been in active development since 2016. The goal of the project is to provide users with everything that is needed to build rich and engaging web applications using the Material Design specification.
They also provide support so if you are really stuck you can book a consultant online and discuss your problem.
Vuetify Version 2
Version 2 has some breaking changes in compare to version 1 that you might consider it during installation.
Steps to setup Vuetify in JSS Vue based app
- Install vuetify library
- Install sassLoader and vuetify loader
- Injected vuetify to Vue instance
- Override vuetify variables and brand colors
- Add
as a required element - Configured sass-loader to inject variables once Vuetify is loaded
- Used VuetifyLoaderPlugin for tree-shaking
Install vuetify and all loaders
Basically you can install either a plugin which takes care of a few things for you or install libraries manually. Install vue-cli-plugin-vuetify by adding plugin to your Vue app. To add it, you can run Vue cli UI and add plugin through graphic UI.
If you are manually install it, run these commands or look at Vuetify doc
npm install vuetify (version 2+)
npm install sass sass-loader vuetify-loader
Introduce Veutify to your app
You can create a folder for Vuetify and inside the index.js
file import your Vuetify library. Also you can define your brand colors in a js file as well and inject it to Vuetify.
import Vue from 'vue';
import Vuetify from 'vuetify/lib';
import colors from "./colors";
Vue.use(Vuetify);
const opts = {
theme: {
themes: colors
}
}
export default new Vuetify(opts);
A sample of color.js
file is like this:
const colors = {
"light-gray": "#F5F5F5",
"green": "#48A23F",
"red": "#D32F2F",
};
export default colors;
Then you can inject it to a Vue
instance while you are instantiating it.
In pure Vue app built by Vue-cli you can do it in main.js
file but when you build your app with JSS
then use the CreateApp.js
file:
**import vuetify from './vuetify';**
export function createApp(initialState, i18n) {
Vue.config.productionTip = false;
const router = createRouter();
const graphQLProvider = createGraphQLProvider(initialState);
const vueOptions = {
apolloProvider: graphQLProvider,
router,
**vuetify**,
render: (createElement) => createElement(AppRoot),
};
// conditionally add i18n to the Vue instance if it is defined
if (i18n) {
vueOptions.i18n = i18n;
}
const app = new Vue(vueOptions);
// if there is an initial state defined, push it into the store, where it can be referenced by interested components.
if (initialState) {
app.$jss.store.setSitecoreData(initialState);
}
return { app, router, graphQLProvider };
}
Kick off Vuetify by adding
v-app
is a required element and you need to add it to the first level of vue template. In pure Vue application just add it in to App.vue
but if you use JSS
then add it to your Layout.vue
file.
Override default Vuetify variables
Vuetify version1
used to work with Stylus which was bit of a problem; However, in version 2
they moved to Sass which is more comfortable and consistent for me.
You need to build your own Style folder and define the variable.scss
file.
Then you need to inject it to Vuetify while it is loading which needs vue.config.js
or webpack.js
if you use it directly.
In Vue.config.js
add these lines:
let vueConfig = {};
//Inject the custome scss file to vuetify to override default variables
vueConfig.css= {
loaderOptions: {
scss: {
data:"@import '@/Styles/variable.scss'"
}
}
}
Tree shaking
If you want to drop the unused components in your build then use vuetify-loader/lib/plugin
plugin.
const VuetifyLoaderPlugin = require('vuetify-loader/lib/plugin');
vueConfig.configureWebpack = (config) => {
config.plugins.push(new VuetifyLoaderPlugin());
}
module.exports = vueConfig;
``